Clients & Servers & Sockets, Oh My!
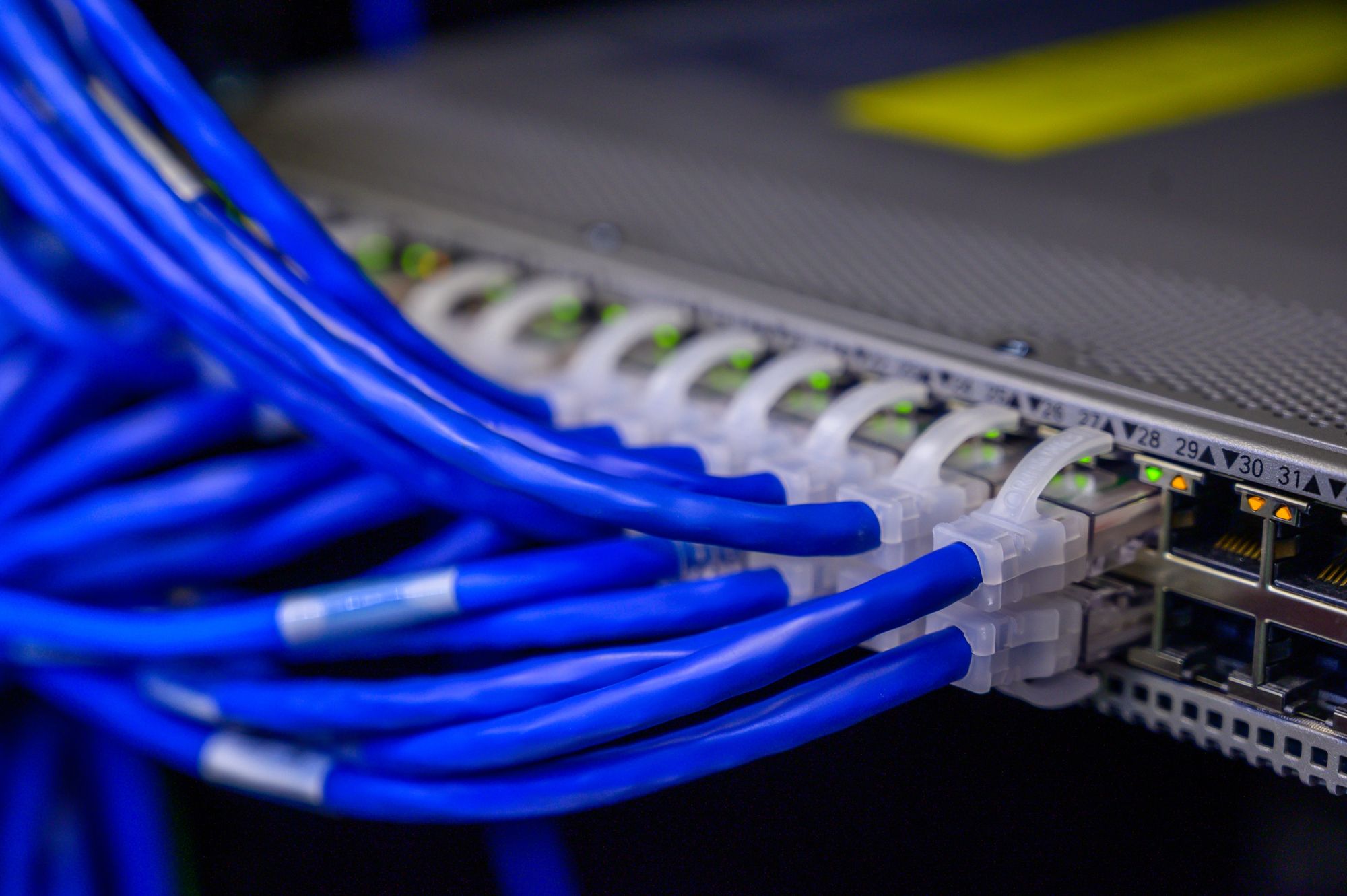
Thus far the Practical Ethical Hacking course - after advising anyone watching to have some basic network knowledge - has really taken its time walking students through each topic. Halfway through the Python module of the course it finally hit a point where an understanding how clients and servers communicate would be helpful. For anyone interested in taking this course that hasn't had much exposure to networking principals, let me give you some highlights about clients, servers, and sockets, so that you can get through this module of the class understanding everything that happened.
The Client-Server Model
The first quick concept here is called the client-server model. Fairly straightforward; there is a client ( which is the user - aka you - and whatever application you're running ), and there is a server that the client is trying to connect to and get information from. That's the basic gist of the client-server model. Client connects to server and asks for something, server responds.
If you want to, you can think of this as if it were two people having a conversation. Just as in real life, the person initiating the conversation ( the client ) needs to find the person that they want to talk to ( the server), and that person has to be willing to listen. Let's tackle the client first:
The Client
The client needs to find the server that it wants to talk with, to do this it needs two things; an IP address and a port number.
Think of the IP address as the location of the server on the internet, its home address if an analogy helps.
Once you have an IP address and you've made your way to where the server is at, you need only to knock on the door - except this is actually a massive apartment building. There are literally thousands of doors; you've got some time to kill so you count them all and find there are exactly 65,535. How do you know which one to knock on? Here is where the port number comes in to play, this number represents the door behind which the server is waiting and listening for a knock.
When you combine an IP address and port number into a single item - an "object" - you have a socket. With this socket in hand, the client is ready to connect with the server.
The Server
This is going to be much shorter then the client section, really the client has done all the work. The server is just waiting at home for someone to come knocking. When a client finds the server (using its IP address), and knocks on the right door (using the port number), the server responds by accepting the connection - basically opening the door - and then handing over what was requested.
The Weeds
If that made sense to you then you probably have enough understanding to make it as far as I've made it in the course. We'll see how much more complicated the networking gets as the course progresses, so far though the presenter really has taken care to explain what's been happening - mostly this post is just adding some context.
This last bit isn't strictly necessary to understand the current Python module, but if you want a little something extra here it is.
This module has an assignment to follow along as the presenter writes a simple port scanner, and there are some Python commands and syntax I can explain. The presenter goes over some of this, but a little repetition while learning never hurts. Here we go:
- socket.gethostbyname(): This function accepts a value, either an IP address or a hostname ( www.google.com would be an example of a hostname ). If an IP address is passed in then it returns that same string, if a hostname is passed in then it finds that host's IP address and returns that value.
- socket.socket( socket.AF_INET, socket.SOCK_STREAM ): This one is a bit of a mouth-full. What's happening here is a new socket object is being created. We can see this object has the parameters of socket.AF_INET and socket.SOCK_STREAM.
This first parameter, socket.AF_INET, is telling this newly created socket that it needs to be ready to take in an IPv4 IP address.
The second parameter, socket.SOC_STREAM, tells the socket that its second value will be an integer representing a port number.
- socket.connect_ex( address ): This function is used by the client to connect to the server. The address parameter is actually a tuple that contains both the IP address of the sever, as well as the port number it's listening on. Lastly, if a connection attempt is successful then this function will return a zero. If anything other than a zero gets returned, the connection has failed and the returned number identifies the particular error.
Closing
That should be plenty to make sure you can follow along with the Python module, at least as far as the Port Scanner assignment - which is as far as I've made it. Hopefully this has been helpful to anyone else taking the course. If...more likely when...this course wades further into the weeds of networking I'll make another post.
References
Python 3.11.3 Documentation - Additional details on socket objects and the functions used.
Client-Server Model - GeeksforGeeks for information about the client-server model.
Python - Get IP Address from Hostname - DigitalOcean for a deeper dive into the gethostbyname function.